Introduction to Jupyter-notebooks and Python primer¶
Python is a modern complete an open source programming language
Widespread in the scientific community
Easy to understand and human-readable
Still C wins on speed - but python is versatile and has packages that compete with C (such as NumPy)
See an extended python tutorial here: https://docs.python.org/3/contents.html
How to use Jupyter-notebook¶
Each cell can be run independently, regardless of the order
Intersting short-cuts:
Press shift+Enter to run a cell
Press ESC+D to delete a cell
Press ESC+A to create a cell above your current cell
Press ESC+B to create a cell below your current cell
Programming basics with Python¶
Indentation¶
Python uses indentation for blocks, instead of curly braces. Both tabs and spaces are supported, but the standard indentation requires standard Python code to use four spaces. For example:
[1]:
x=1
if x==1:
print("x is 1.")
x is 1.
Variables and types¶
Python is completely object oriented, and not “statically typed”. You do not need to declare variables before using them, or declare their type. Every variable in Python is an object.
Numbers¶
Python supports two types of numbers - integers and floating point numbers.
[2]:
myint=7 # this is an integer
print(myint)
7
[3]:
myfloat = 7.0 # this is a float number
print(myfloat)
7.0
Strings¶
Strings are defined either with a single quote or a double quotes. The difference between the two is that using double quotes makes it easy to include apostrophes (whereas these would terminate the string if using single quotes)
[4]:
mystring = 'hello'
print(mystring)
mystring = "hello"
print(mystring)
hello
hello
Simple operators can be executed on numbers and strings:
[5]:
one = 1
two = 2
three = one + two
print(three)
hello = "hello"
world = "world"
helloworld = hello + " " + world
print(helloworld)
3
hello world
Assignments can be done on more than one variable “simultaneously” on the same line like this
[6]:
a, b = 3, 4
print(a,b)
3 4
Mixing operators between numbers and strings is not supported:
[7]:
# This will not work!
one = 1
two = 2
hello = "hello"
#print(one + two + hello)
Lists¶
Lists are very similar to arrays. They can contain any type of variable, and they can contain as many variables as you wish. Lists can also be iterated over in a very simple manner. Here is an example of how to build a list.
[8]:
mylist = []
mylist.append(1)
mylist.append(2)
mylist.append(3)
print(mylist[0]) # prints 1
print(mylist[1]) # prints 2
print(mylist[2]) # prints 3
1
2
3
To see all lists built-in methods: https://docs.python.org/3/tutorial/datastructures.html
Standard arithmetical operations¶
[9]:
a=3+4
print(a)
7
[10]:
b=25-20
print(b)
5
[11]:
c=30*2
print(c)
60
[12]:
d=2**3 # Exponentiation (everything written after '#' is a comment and is ignored by the interpreter)
print(d)
8
Another operator available is the modulo (%) operator, which returns the integer remainder of the division. dividend % divisor = remainder.
[13]:
remainder = 11 % 3
print(remainder)
2
Using operators with lists:
[14]:
even_numbers = [2,4,6,8]
odd_numbers = [1,3,5,7]
all_numbers = odd_numbers + even_numbers
print(all_numbers)
[1, 3, 5, 7, 2, 4, 6, 8]
String formatting and basic string operations¶
You can print variables within strings. Add the tag f” before the string and insert the variables in curly brackets: {variable}
[15]:
print(f"The list of even numbers is {even_numbers}")
The list of even numbers is [2, 4, 6, 8]
[16]:
my_string="Today is Monday"
You can perform several operations on strings:
[17]:
# Such as obtaining the length:
print(len(my_string))
15
[18]:
# counting the number of times a letter appears:
print(my_string.count("o"))
2
[19]:
# you can slice the string:
print(my_string[2:5])
print(my_string[2:15:1])
print(my_string[2:15:2])
#This prints the characters of string from 3 to 7 skipping one character.
#This is extended slice syntax. The general form is [start:stop:step].
day
day is Monday
dyi ody
[20]:
# you can reverse a string:
print(my_string[::-1])
yadnoM si yadoT
See other string methods here: https://docs.python.org/3/library/string.html#module-string
Conditions¶
Python uses boolean variables to evaluate conditions. The boolean values True and False are returned when an expression is compared or evaluated. For example:
[21]:
x = 2
print(x == 2) # prints out True
print(x == 3) # prints out False
print(x < 3) # prints out True
True
False
True
Notice that variable assignment is done using a single equals operator “=”, whereas comparison between two variables is done using the double equals operator “==”. The “not equals” operator is marked as “!=”.
Boolean operators¶
The “and” and “or” boolean operators allow building complex boolean expressions, for example:
[22]:
name = "John"
age = 23
if name == "John" and age == 23:
print("Your name is John, and you are also 23 years old.")
if name == "John" or name == "Rick":
print("Your name is either John or Rick.")
Your name is John, and you are also 23 years old.
Your name is either John or Rick.
The “in” operator¶
The “in” operator could be used to check if a specified object exists within an iterable object container, such as a list:
[23]:
name = "John"
if name in ["John", "Rick"]:
print("Your name is either John or Rick.")
Your name is either John or Rick.
The “is” operator¶
Unlike the double equals operator “==”, the “is” operator does not match the values of the variables, but the instances themselves. For example:
[24]:
x = [1,2,3]
y = [1,2,3]
print(x == y) # Prints out True
print(x is y) # Prints out False
True
False
The “not” operator¶
Using “not” before a boolean expression inverts it:
[25]:
print(not False) # Prints out True
print((not False) == (False)) # Prints out False
True
False
Loops¶
the for loop¶
For loops iterate over a given sequence. Here is an example:
[26]:
primes = [2, 3, 5, 7]
for prime in primes:
print(prime)
2
3
5
7
For loops can iterate over a sequence of numbers using the “range” and “xrange” functions. The difference between range and xrange is that the range function returns a new list with numbers of that specified range, whereas xrange returns an iterator, which is more efficient. (Python 3 uses the range function, which acts like xrange). Note that the range function is zero based.
[27]:
# Prints out the numbers 0,1,2,3,4
for x in range(5):
print(x)
# Prints out 3,4,5
for x in range(3, 6):
print(x)
# Prints out 3,5,7
for x in range(3, 8, 2):
print(x)
0
1
2
3
4
3
4
5
3
5
7
while loops¶
[28]:
# Prints out 0,1,2,3,4
count = 0
while count < 5:
print(count)
count += 1 # This is the same as count = count + 1
0
1
2
3
4
break and continue statements¶
break is used to exit a for loop or a while loop, whereas continue is used to skip the current block, and return to the “for” or “while” statement. A few examples:
[29]:
# Prints out 0,1,2,3,4
count = 0
while True:
print(count)
count += 1
if count >= 5:
break
# Prints out only odd numbers - 1,3,5,7,9
for x in range(10):
# Check if x is even
if x % 2 == 0:
continue
print(x)
0
1
2
3
4
1
3
5
7
9
Functions¶
Functions are a convenient way to divide your code into useful blocks, allowing us to order our code, make it more readable, reuse it and save some time. Also functions are a key way to define interfaces so programmers can share their code. Functions in python are defined using the block keyword “def”, followed with the function’s name as the block’s name. For example:
[30]:
def my_function():
print("Hello From My Function!")
[31]:
# Now we can call the function
my_function()
Hello From My Function!
Let’s make something more interesting. Python functions also receive arguments:
[32]:
def sum_two_numbers(a, b):
return a + b
[33]:
sum_two_numbers(5,12)
[33]:
17
Dictionaries¶
A dictionary is a data type similar to arrays, but works with keys and values instead of indexes. Each value stored in a dictionary can be accessed using a key, which is any type of object (a string, a number, a list, etc.) instead of using its index to address it.
For example, a database of phone numbers could be stored using a dictionary like this:
[34]:
phonebook = {}
phonebook["John"] = 938477566
phonebook["Jack"] = 938377264
phonebook["Jill"] = 947662781
print(phonebook)
{'John': 938477566, 'Jack': 938377264, 'Jill': 947662781}
[35]:
phonebook = {"John" : 938477566,"Jack" : 938377264,"Jill" : 947662781}
for name, number in phonebook.items():
print("Phone number of %s is %d" % (name, number))
Phone number of John is 938477566
Phone number of Jack is 938377264
Phone number of Jill is 947662781
Modules and packages¶
Modules in Python are simply Python files with a .py extension. They can also be packages that contain several .py files. The name of the module will be the name of the file or package. A Python module can have a set of functions, classes or variables defined and implemented.
Modules are imported using the import command. We will use the protlego module through these tutorials
[36]:
from protlego import *
Plots using matplotlib module¶
[37]:
from matplotlib.pylab import plot,title,xlabel,ylabel,grid,show,savefig,gcf
import numpy as np
#Optional: magic line to make plots inline
%matplotlib inline
x = np.arange(0,50,.5)
y = np.sin(x/3) - np.cos(x/5)
plot(x,y, '.-k')
plot(x,np.sin(x/2) - np.cos(x/5),'.r')
title('A simple double plot')
xlabel('variable 1'), ylabel('variable 2'), grid(True)
fig = gcf() # Get current figure
show()
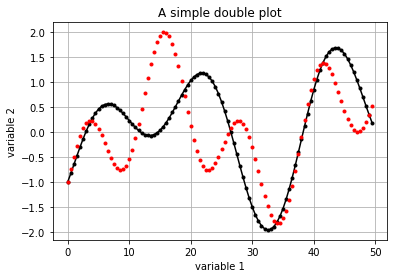
Getting help¶
[38]:
help(show)
Help on function show in module matplotlib.pyplot:
show(*args, **kw)
Display a figure.
When running in ipython with its pylab mode, display all
figures and return to the ipython prompt.
In non-interactive mode, display all figures and block until
the figures have been closed; in interactive mode it has no
effect unless figures were created prior to a change from
non-interactive to interactive mode (not recommended). In
that case it displays the figures but does not block.
A single experimental keyword argument, *block*, may be
set to True or False to override the blocking behavior
described above.
Some exercises¶
Sum the first 50 numbers with a for loop
[ ]:
Write a Python function to display the first and last items from a list.
[ ]:
Write a Python function to concatenate all elements in a list into a string and return it.
[ ]: